Dehlila
Phone/SMS Therapy
The project began with the discovery of the emacs application titled Doctor. This application exists on every mac and allows the user to type phrases and sentences into the Terminal generating a response hypothetically from a therapist. The application is originally based on an application titled Eliza which was developed in the 1960′s. The program functions on a series of pattern matching algorithms which lead the user to believe that the program is alive. Eliza in fact analyzes each word from the users and responds with predetermined response including several words from the user.
For my final project, I decided to create a phone service that would allow a user to verbally converse with the Eliza application. A users verbal input is sent into Google’s voice recognition API which then sends the transcribed results into the Eliza application. The Eliza application would then generate a text response which is then passed into Google’s text-to-speech API. This is then sent back to the user in the form of a computer voice.
To develop the application, I began working in Asterisk to setup a dial plan which would receive phone calls and execute other actions. Once a call has reached the dial plan, the user is greeted by a robot voice, through Google text-to-speech, which asks the user what they would like to talk about. The users is then allowed to say whatever they would like after the beep which is sent directly into Google’s Voice recognition API. This API returns a variable which can be accessed in the dial plan.
Originally, I worked towards passing this variable through an AGI script in Asterisk in order to generate a response from Eliza. This method was unsuccessful and ultimately unnecessary. Instead, I used the SHELL command inside of Asterisk to run a ruby script of Eliza while also passing the returned variable from Google’s Voice API into the ruby script. The result of this was then passed back into the dial plan as a new variable. Here is the script.
Set(eliza_reply=${SHELL(ruby /home/nejohnson2/asterisk_agi/ElizaRuby_update/eliza2.rb "${utterance}")})
From here, the new variable resulting from the Eliza script, is passed into an AGI script which executes the Google text-to-speech service.
AGI(googletts.agi, "${eliza_reply}",en)
And that it! After these two commands are executed, the call is sent back to the beginning of the dial plan in which it begins listening to the caller for a new response. This loop continues endlessly until the call is ended by the user.
[mstts]
exten => s,1,AGI(googletts.agi, "hello, My name is Eliza, and I am a computer psychotherapist. What do you want to talk about?",en)
exten => s,2,agi(speech-recog.agi,en-US)
exten => s,n,noop(${utterance})
exten => s,n,Set(eliza_reply=${SHELL(ruby /home/nejohnson2/asterisk_agi/ElizaRuby_update/eliza2.rb "${utterance}")})
exten => s,n,AGI(googletts.agi, "${eliza_reply}",en)
exten => s,n,noop(${eliza_reply})
exten => s,n,Verbose(1,The text you just said is: ${utterance})
exten => s,n,Verbose(1,The probability to be right is: ${confidence})
exten => s,n,Wait(1)
exten => s,n,GoTo(mstts,s,2)
This version of Eliza however was very difficult to interact with from a user perspective. This was primarily due to limitations within the technology. Using Google’s Voice recognition was easy to implement but often does not return accurate results. In order to get Google Voice recognition to work well, the user must be in a noiseless room and speak slowly and clearly. Even under these scenarios however, results varied. The other difficulty in working with Google Voice recognition is the fact that it needs two seconds of silence to signal to the API that the user is done speaking. This completely disrupts the flow of a normal conversation and ultimately frustrates the user.
As a second iteration of this project, I decided to transform the voice version into a texting service. A user could send an SMS to Eliza who would then return an SMS. This would eliminate using voice recognition to generate a response causing the application to be much more accurate and responsive.
To begin the texting service, I setup an account with Twilio which would handle receiving and sending SMS messages to the user. When Twilio receives an SMS, it makes a post request sending To, From and message body information. With this information, I created a Sinatra application which would receive the body of the SMS and pass it into the ruby version of Eliza. This code was much more simple to work with.
post '/' do @account_sid = 'XXXXXXXXXXXXX' @auth_token = 'XXXXXXXXXXXXX' @client = Twilio::REST::Client.new(@account_sid, @auth_token) @account = @client.account # .first grabs the newest message sent # .body grabs the body of the newest sms $utterance = @account.sms.messages.list({:to => '16464309130'}).first.body # Execute the eliza app Eliza.run(Rule::ELIZA_RULES2) # $Eliza_Response is the response from the eliza app # execute XML to send a response back to the caller content_type 'text/xml' "Response>Sms>#{$Eliza_Response}/Sms>/Response>" end
The first four lines of this script are setting making the connection to the my Twilio account. The following line grabs the most recent text message and parses out the body of the message setting to the variable $utterance. The post request then executes the Eliza ruby script with the variable $utterance returning the response to a new variable $Eliza_Response. This variable is then placed into XML which Twilio interprets as a send SMS message back to the user. The body of this message being Eliza’s response.
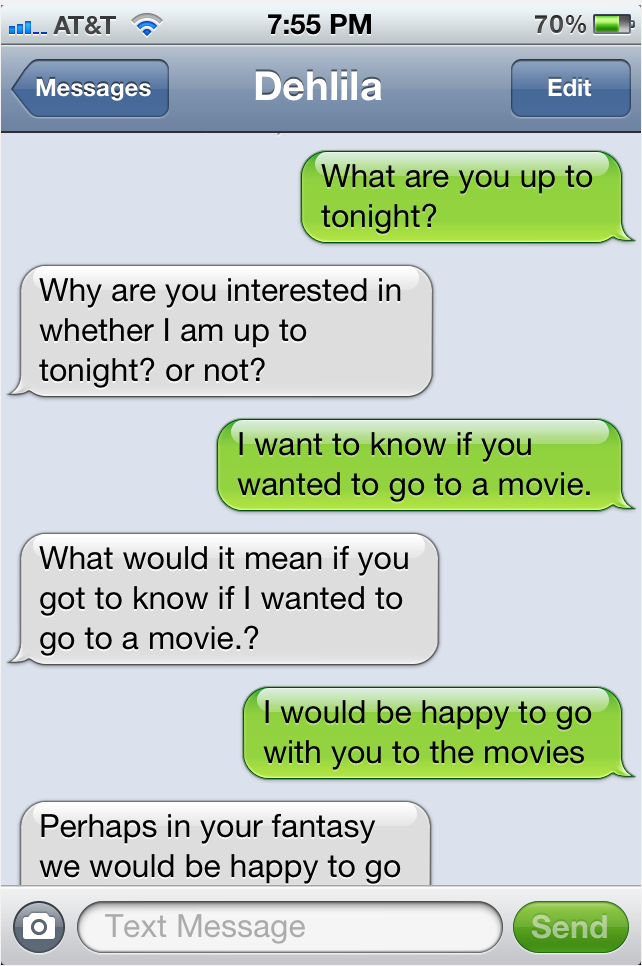
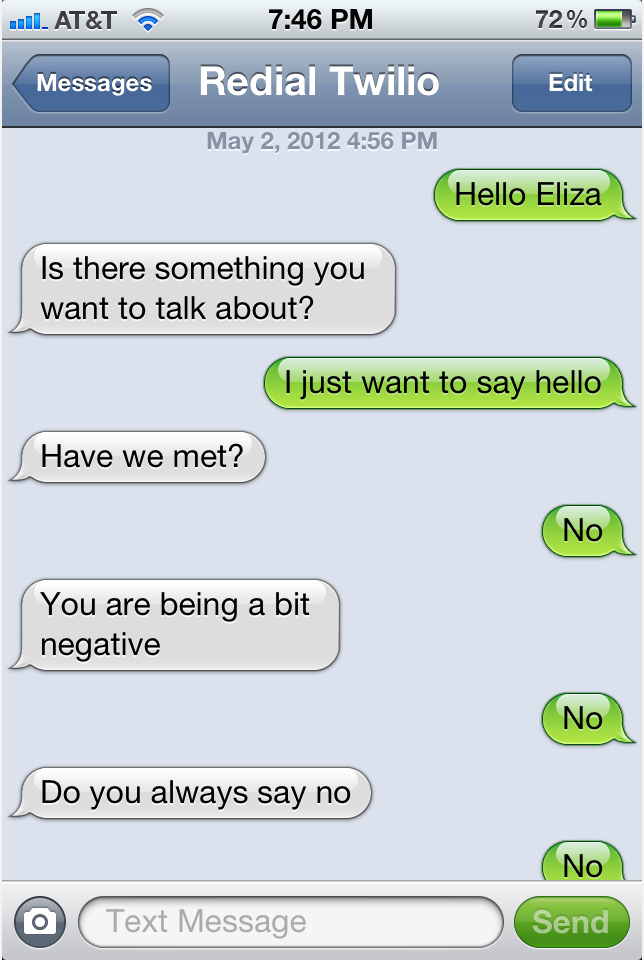
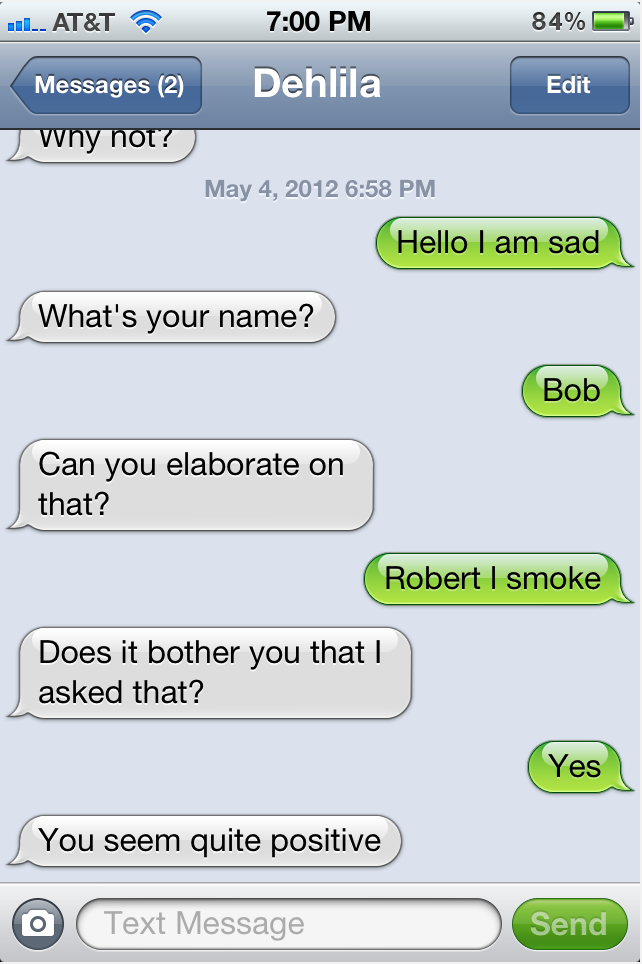